Bash Scripting for Beginners - A Practical Guide
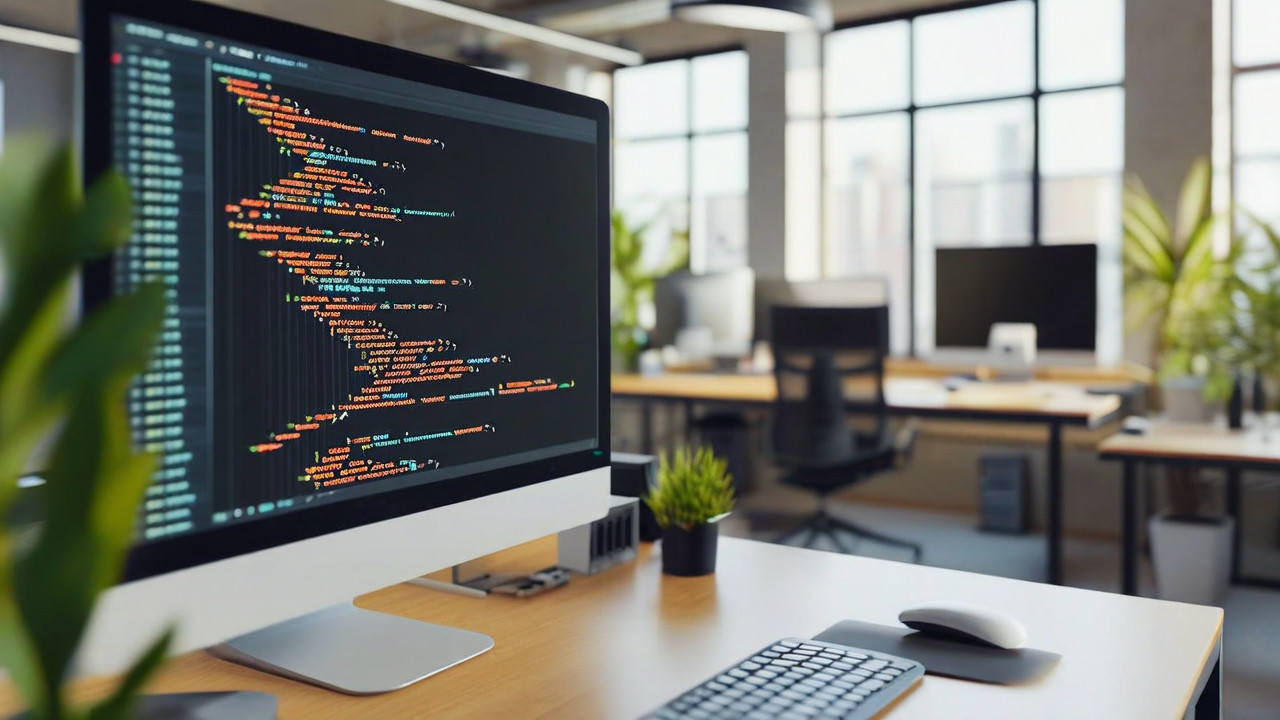
Bash Scripting for Beginners - A Practical Guide
Bash scripting is a powerful tool that allows you to automate tasks and create efficient workflows in Linux and Unix-like systems. In this guide, we’ll cover the basics of bash scripting to help you get started.
What is Bash Scripting?
Bash (Bourne Again SHell) is a command-line interpreter that allows you to execute commands and create scripts. A bash script is simply a text file containing a series of commands that are executed in sequence.
Getting Started
1. Creating Your First Script
Let’s create a simple “Hello World” script:
#!/bin/bash
echo "Hello, World!"
Save this as hello.sh
. The first line #!/bin/bash
is called a shebang and tells the system to use bash to interpret the script.
2. Making the Script Executable
Before running your script, you need to make it executable:
chmod +x hello.sh
3. Running the Script
You can run your script in two ways:
./hello.sh
# or
bash hello.sh
Basic Bash Scripting Concepts
Variables
Variables in bash are created by assigning values:
name="John"
age=25
To use a variable, prefix it with $
:
echo "My name is $name and I am $age years old"
User Input
You can get input from users using the read
command:
echo "What's your name?"
read name
echo "Hello, $name!"
Conditional Statements
Bash supports if-else statements:
if [ $age -gt 18 ]; then
echo "You are an adult"
else
echo "You are a minor"
fi
Loops
For Loop
for i in {1..5}; do
echo "Number: $i"
done
While Loop
count=1
while [ $count -le 5 ]; do
echo "Count: $count"
((count++))
done
Practical Example: File Backup Script
Let’s create a simple backup script:
#!/bin/bash
# Create backup directory if it doesn't exist
mkdir -p ~/backups
# Create backup filename with date
backup_file="backup_$(date +%Y%m%d).tar.gz"
# Create backup
tar -czf ~/backups/$backup_file ~/Documents
echo "Backup created: $backup_file"
Best Practices
- Always start your scripts with
#!/bin/bash
- Use meaningful variable names
- Add comments to explain complex operations
- Test your scripts thoroughly
- Handle errors appropriately
Additional Resources
To help you further master Bash scripting, here are some excellent video tutorials:
1. Bash Scripting for Beginners
2. Advanced Bash Scripting
3. Bash Scripting Best Practices
Conclusion
Bash scripting is a valuable skill that can help you automate tasks and improve your productivity. Start with simple scripts and gradually build more complex ones as you become comfortable with the basics.
Remember: Practice makes perfect! Try creating your own scripts and experiment with different commands and structures.
Happy scripting! 🚀
Share Your Thoughts!
If you found this guide helpful, please show your support by clicking the reaction buttons below (👍, ❤️, or 🎉). Your feedback helps me create better content!
Comments